Software development
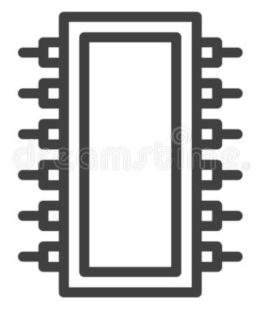
A simple and inexpensive shift register can be used to increase the digital output provision of a Raspberry Pi or microcontroller. This well-know technique is easy to apply, but has some limitations that require careful consideration.
Categories: software development, C, embedded computing, Raspberry Pi
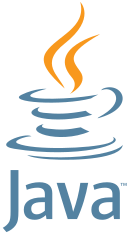
This article extends my earlier article on using Avro with a message broker. In this article I explain how to use the Apicurio schema registry to store Avro schema artefacts that are shared by multiple clients, rather than providing local copies for each client.
Categories: software development, Java, middleware
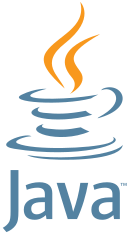
This article describes how to use Apache Avro to flatten a Java object, pass it through a JMS-compatible message broker, and reconstruct it again. Avro is a compact, schema-based data representation that is becoming increasingly important in messaging applications.
Categories: software development, Java, middleware
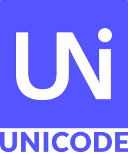
There's been a recent scare that Unicode reading direction characters could be used to conceal malicious code in open-source projects. This is undoubtedly true, but that fact doesn't make it significantly harder to ensure the security of open-source code than it already is.
Categories: software development, security
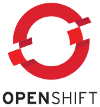
Although Java dominates the webservices world, there are gains to be made by considering the use of lower-level lanaguages like C, particularly in a containerized, microservice architecture. This article describes one way to implement a webservice for Red Hat OpenShift, and similar systems, using C and an ultra-lightweight container base image.
Categories: software development, OpenShift
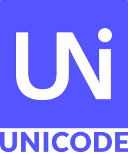
Java's strict adherence to Unicode standards for text representation ought to make life easier for developers, and usually it does. But not always.
Categories: software development
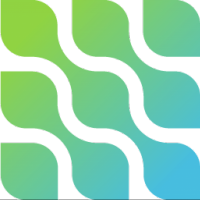
The notion of change data capture (CDC) is becoming increasingly significant, in an IT industry that stores and manages an ever-increasing volume of data. This article describes how to begin using the Debezium CDC framework with Apache Came, to collect and process change records from disparate data sources.
Categories: software development, Java, middleware
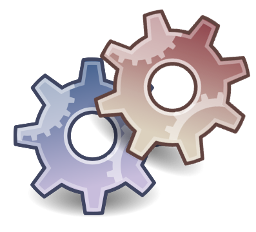
This article describes how to write a trivial program for Linux using no compiler tools at all, but entering machine language directly in hexadecimal. Because we can.
Categories: software development, Linux
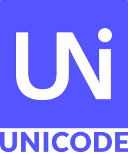
How character encoding actually works, and the implications for developers of choosing one character set over another.
Categories: software development
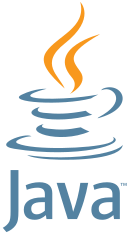
Running a shell script from a Java program using Runtime.exec()
appears simple. In practice, there are many pitfalls. This article describes how to avoid at least some of them.
Categories: software development, Java
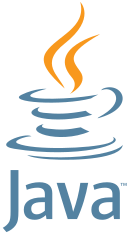
A quick-and-dirty way to make it possible to execute Java JAR files at the prompt, without needing to invoke the JVM.
Categories: software development, Java, Linux
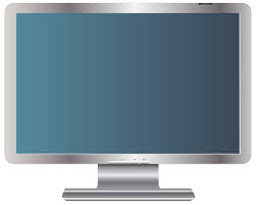
Writing graphical applications for minimal and embedded Linux systems can present a challenge. One of the problems is producing nicely-rendered text without the facilities that a graphical desktop would provide. This article describes how to use the FreeType library to render text to the Linux framebuffer.
Categories: software development, C, Linux, embedded computing, Raspberry Pi
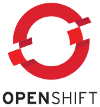
This article describes in detail the steps the Fabric8 Maven plug-in carries out when deploying an application to OpenShift.
Categories: software development, Java, OpenShift
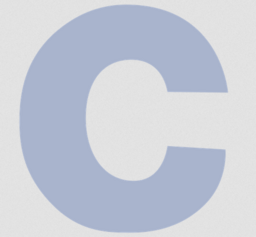
Sometimes it's helpful to be able to create an executable program that embeds all the data it needs, and provide that data as files. C/C++ do not provide any standard way to do this, but GCC has facilities that the developer can use.
Categories: software development, C
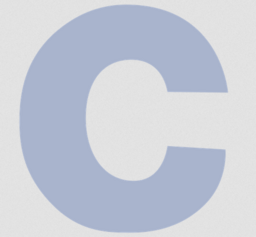
I see too many C (and C++) programs misbehave at runtime, for reasons that could easily have been detected using checks built into all modern compilers. This article describes some common C programming errors, and shows how they would have been spotted easily if the compiler were configured correctly.
Categories: software development, C
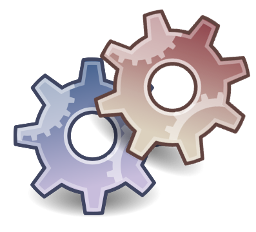
The GraalVM 'Native Image' plugin has the capability to compile to stand-alone binaries languages like Java that are normally compiled on the fly. This article describes step-by-step how to install GraalVM and the plug-in, and use it on some simple Java examples.
Categories: software development, Java
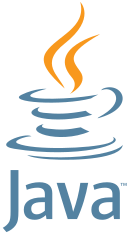
Functional interfaces are allow Java programmers to write methods whose arguments are lambda functions. How does this work, and why would it be useful?
Categories: software development, Java
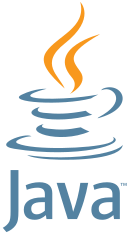
Many of the standard Java APIs make extensive use of anonymous inner classes, to provide features that in other language would be provided using lambda functions or closures. However, anonymous inner classes have limitations that many developers find difficult to understand and to work with. This article explains why this is the case.
Categories: software development, Java
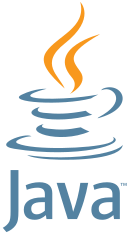
Why does Java have a NullPointerException when it doesn't support pointers? Why does the equality operator compare object references, but the collections framework comparison methods compare object contents? These questions and many more like them will comprehensively fail to be answered in this article.
Categories: software development, Java
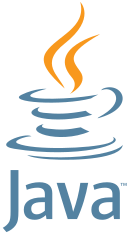
Developers don't use @Override enough. Java's method visibility rules, when classes are in different packages, make polymorphic inheritance behave in odd ways. Careful use of @Override can prevent these problems, and make applications much easier to maintain.
Categories: software development, Java
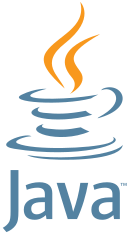
New auto-compilation features in Java 11 give Java the potential to behave more like a traditional scripting language, such as Perl or Python. But how useful are these features in practice?
Categories: software development, Java
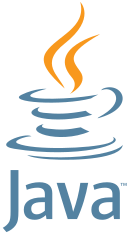
The first part in a series on concurrency management in multi-threaded Java programs. This article deals with monitor objects and their use for demarcation of non-concurrent sections.
Categories: software development, Java

Although the Java Media Framework can do some very sophisticated things, it remains relatively difficult to generate simple musical tones using Java. The de-facto method for specifying musical notes remains the MIDI file, although there are now more sophisticated approaches. It's surprisingly difficult to generate even a simple MIDI file in Java, not least because of the lack of unsigned data types. I wrote this article years (perhaps decades) ago, but it's recently become popular again, because of the use of Java in Android, and the lack of any Java API in Android for playing musical tones, other than by reading a MIDI file.
Categories: software development, music, Java

A quick-start guide that highlights the similarities and differences between the Java and Perl programming languages.
Categories: software development, Java, Perl
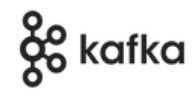
Kafka Streams is a Java library and framework for creating applications that consume, process, and return Apache Kafka messages. This article provides a tutorial about implementing a very basic Streams application.
Categories: software development, Java
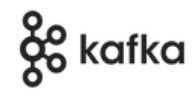
Following on from my article on the rudiments of the Kafka Streams API, this one introduces stateful operations like counting and aggregation.
Categories: software development, Java
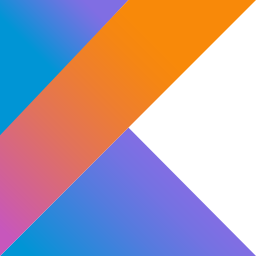
Kotlin is a Java-compatible programming language that has increased in popularity very quickly over the last few years. This article provides the briefest of overviews, for experienced Java developers who might be considering using Kotlin.
Categories: software development, Java
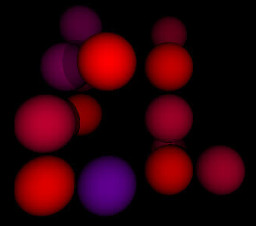
Implementing a program to run Conway's cell population simulation, using a 3D perspective view on the Linux framebuffer.
Categories: software development, C, Linux
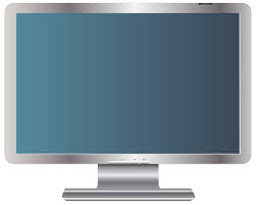
The absolute minimum information needed to start using the Linux framebuffer as a graphical display in C/C++ applications.
Categories: software development, C, Linux, embedded computing
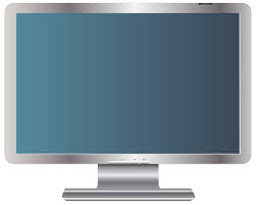
This article continues my original framebuffer just the essentials article, by describing how to handle less straightforward framebuffer configurations.
Categories: software development, C, Linux, embedded computing
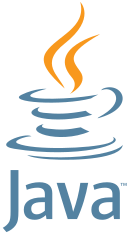
What can the software industry learn from the Log4J security debacle?
Categories: software development, Java, security
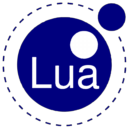
The essentials of Lua programming in one page, for experienced Java programmers.
Categories: software development, Java, Lua
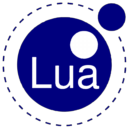
Lua is an embeddable scripting language, which can be extended in a number of useful ways. This article describes in detail how to create a Lua extension in C (or, with a bit of fiddling, C++) as a loadable (.so) library.
Categories: software development, Lua, C
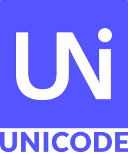
How an oddity in the way UTF-8 encoding works can cause all sorts of problems for unwary developers, including security weaknesses.
Categories: software development, security
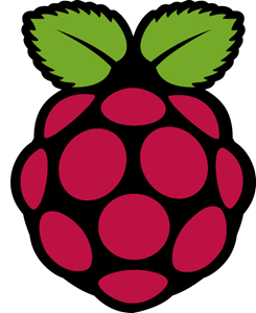
A series of simple, progressive examples that demonstrate the essential features of programming in ARM assembly language.
Categories: Raspberry Pi, software development, embedded computing, assembly
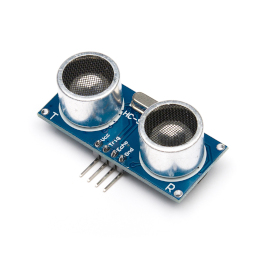
The HC-SR04 proximity sensor is an inexpensive and widely-used ultrasonic device. Connecting one to an HC-SR04 to a Raspberry Pi is a common educational exercise, but getting accurate, repeatable measurement of distance in a real application is actually quite difficult. This article explains why, and what can be done to improve matters.
Categories: software development, C, embedded computing, Raspberry Pi
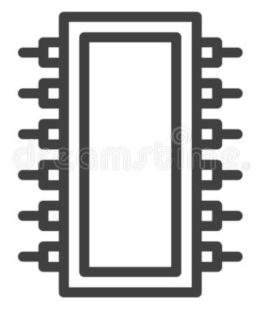
Many battery-backed power supplies for the Raspberry Pi, and similar systems, use the INA219 current/voltage monitor IC. This device has an I2C interface by which the Pi can determine the battery voltage and current, and estimate the charge level and run-time. This article describes how to write C code that interacts with the INA219.
Categories: software development, C, embedded computing, Raspberry Pi
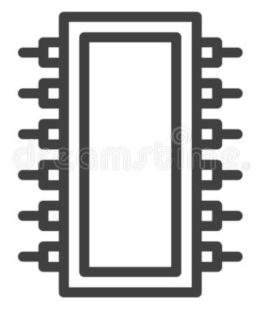
In this article I explain how to construct, and program in C, an I2C interface to the popular HD44780 LCD display for the Raspberry Pi. Between the article and the accompanying source code, no technical details are concealed: I present the complete hardware design and every line of C code needed to operate it.
Categories: software development, C, embedded computing, Raspberry Pi
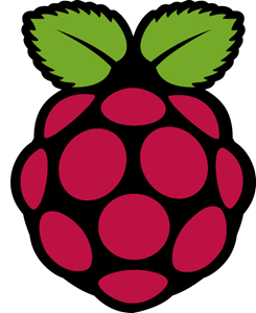
The Raspberry Pi doesn't offer much in the way of analog outputs, or even hardware controlled PWM. Software-controlled PWM is an alternative in some applications, but it needs to be used carefully, if inefficiencies are to be avoided.
Categories: software development, C, embedded computing, Raspberry Pi
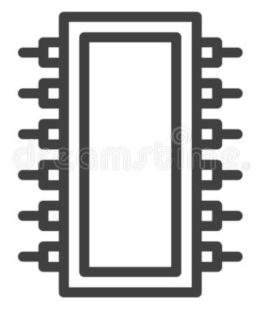
The MAX7219 IC is widely used to control an 8x8 matrix of LED, but they can be chained to create much larger displays. This article describes how the chaining works, and how to create a driver for the Raspberry Pi Pico.
Categories: software development, C, embedded computing, Pico
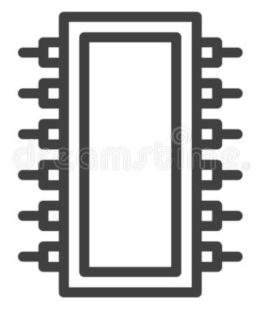
The Pi Pico has USB host support, and can work with a USB keyboard. Although there are some programming examples, the general approach to programming USB host operations is not well documented.
Categories: software development, C, embedded computing, Pico
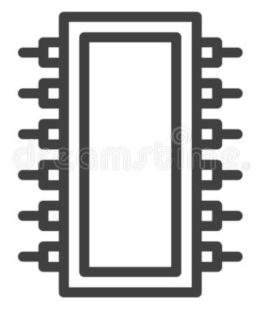
The Pi Pico is an impressive microcontroller for its size and cost, but it lacks specific non-volatile memory. This article explains how to use the program flash ROM for that purpose.
Categories: software development, C, Linux, embedded computing, Pico
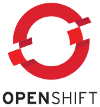
This article describes how to deploy a container image direct from a local repository to an OpenShift 4 cluster, and instantiate a pod based on that image.
Categories: software development, OpenShift
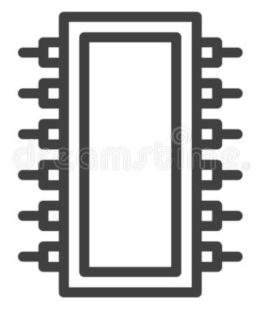
Make an auxiliary LCD display for a computer that displays data sent to it over a USB connection. Ready-made devices of this sort are widely available, but it's more fun to build your own.
Categories: software development, C, Linux, embedded computing, Arduino

Using a Camel application based on Quarkus to provide a way to route messages to and from a message broker using HTTP requests
Categories: software development, Java, middleware

Camel's IRC support makes it relatively easy to implement an IRC conversation agent, that can process IRC messages and produce responses.
Categories: software development, Java, middleware
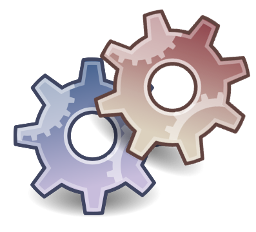
Implementing a webservice in C and Java, to see which performs better in terms of throughput and resource usage.
Categories: software development, Java, C
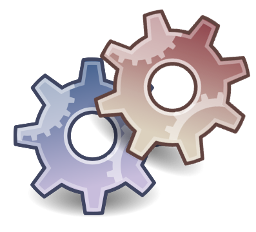
There are relatively few good reasons for writing C code without using a standard C library. However, doing so provides valuable insights into how compilers and operating systems work, and is worth doing if only for its educational value.
Categories: software development, C, embedded computing