Java
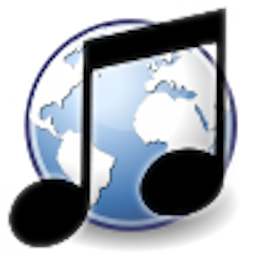
Using an Android device as a remotely-controllable music player.
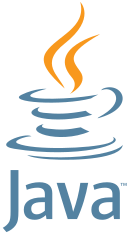
This article extends my earlier article on using Avro with a message broker. In this article I explain how to use the Apicurio schema registry to store Avro schema artefacts that are shared by multiple clients, rather than providing local copies for each client.
Categories: software development, Java, middleware
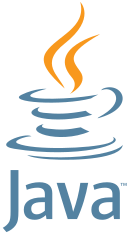
This article describes how to use Apache Avro to flatten a Java object, pass it through a JMS-compatible message broker, and reconstruct it again. Avro is a compact, schema-based data representation that is becoming increasingly important in messaging applications.
Categories: software development, Java, middleware
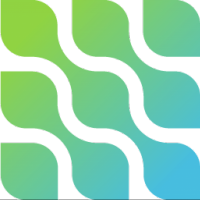
The notion of change data capture (CDC) is becoming increasingly significant, in an IT industry that stores and manages an ever-increasing volume of data. This article describes how to begin using the Debezium CDC framework with Apache Came, to collect and process change records from disparate data sources.
Categories: software development, Java, middleware
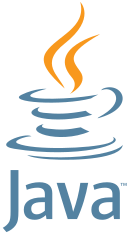
Running a shell script from a Java program using Runtime.exec()
appears simple. In practice, there are many pitfalls. This article describes how to avoid at least some of them.
Categories: software development, Java
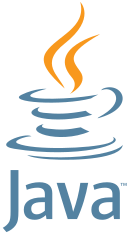
A quick-and-dirty way to make it possible to execute Java JAR files at the prompt, without needing to invoke the JVM.
Categories: software development, Java, Linux
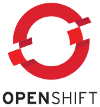
This article describes in detail the steps the Fabric8 Maven plug-in carries out when deploying an application to OpenShift.
Categories: software development, Java, OpenShift
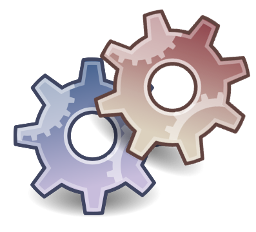
The GraalVM 'Native Image' plugin has the capability to compile to stand-alone binaries languages like Java that are normally compiled on the fly. This article describes step-by-step how to install GraalVM and the plug-in, and use it on some simple Java examples.
Categories: software development, Java
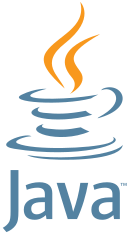
Functional interfaces are allow Java programmers to write methods whose arguments are lambda functions. How does this work, and why would it be useful?
Categories: software development, Java
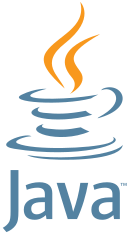
Many of the standard Java APIs make extensive use of anonymous inner classes, to provide features that in other language would be provided using lambda functions or closures. However, anonymous inner classes have limitations that many developers find difficult to understand and to work with. This article explains why this is the case.
Categories: software development, Java
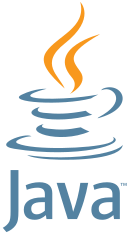
Why does Java have a NullPointerException when it doesn't support pointers? Why does the equality operator compare object references, but the collections framework comparison methods compare object contents? These questions and many more like them will comprehensively fail to be answered in this article.
Categories: software development, Java
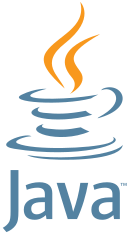
Developers don't use @Override enough. Java's method visibility rules, when classes are in different packages, make polymorphic inheritance behave in odd ways. Careful use of @Override can prevent these problems, and make applications much easier to maintain.
Categories: software development, Java
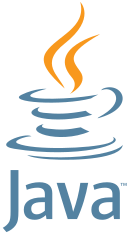
New auto-compilation features in Java 11 give Java the potential to behave more like a traditional scripting language, such as Perl or Python. But how useful are these features in practice?
Categories: software development, Java
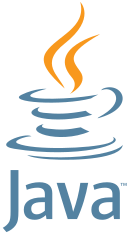
The first part in a series on concurrency management in multi-threaded Java programs. This article deals with monitor objects and their use for demarcation of non-concurrent sections.
Categories: software development, Java

Although the Java Media Framework can do some very sophisticated things, it remains relatively difficult to generate simple musical tones using Java. The de-facto method for specifying musical notes remains the MIDI file, although there are now more sophisticated approaches. It's surprisingly difficult to generate even a simple MIDI file in Java, not least because of the lack of unsigned data types. I wrote this article years (perhaps decades) ago, but it's recently become popular again, because of the use of Java in Android, and the lack of any Java API in Android for playing musical tones, other than by reading a MIDI file.
Categories: software development, music, Java

A quick-start guide that highlights the similarities and differences between the Java and Perl programming languages.
Categories: software development, Java, Perl
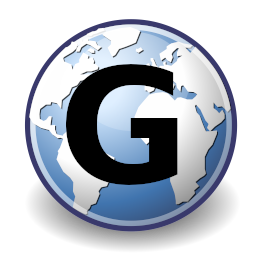
Introducing a simple graphical browser for Project Gemini content; back to the 90s -- in a good way.
Categories: retrocomputing, Java
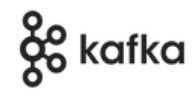
Kafka Streams is a Java library and framework for creating applications that consume, process, and return Apache Kafka messages. This article provides a tutorial about implementing a very basic Streams application.
Categories: software development, Java
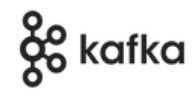
Following on from my article on the rudiments of the Kafka Streams API, this one introduces stateful operations like counting and aggregation.
Categories: software development, Java
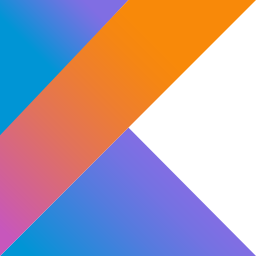
Kotlin is a Java-compatible programming language that has increased in popularity very quickly over the last few years. This article provides the briefest of overviews, for experienced Java developers who might be considering using Kotlin.
Categories: software development, Java
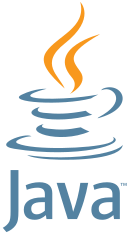
What can the software industry learn from the Log4J security debacle?
Categories: software development, Java, security
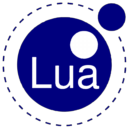
The essentials of Lua programming in one page, for experienced Java programmers.
Categories: software development, Java, Lua

Using a Camel application based on Quarkus to provide a way to route messages to and from a message broker using HTTP requests
Categories: software development, Java, middleware

Camel's IRC support makes it relatively easy to implement an IRC conversation agent, that can process IRC messages and produce responses.
Categories: software development, Java, middleware
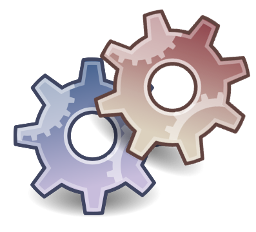
Implementing a webservice in C and Java, to see which performs better in terms of throughput and resource usage.
Categories: software development, Java, C